
Label definition
;**************** Label Definition ********************
cblock h'20'
The data area is automatically assigned from 20h by CBLOCK directive. ENDC is used for the ending of assignment.
The purpose of each data area is shown below.
Label |
| Purpose |
count | : | This area is used for the counter to make 1 second(1Hz), counting a 20-millisecond clock(50Hz) 50 times. |
disp_p | : |
The LED disply position data is stored in this area.
Tens of hour:6, Units of hour:5, Tens of minute:4, Units of minute:3, Tens of second:2, Units of second:1. |
disp_pw | : | This area is the workarea to use when calculating an LED display position. |
disp_data | : | Display data is stored in this area temporarily by the LED display processing. It is used to common-ize the processing of PORTC. |
disp_h10w | : | This area is the workarea to process the contents of the tens of hour. |
disp_h10 | : | The data of the tens of hour is stored in this area. |
disp_h1 | : | The data of the units of hour is stored in this area. |
disp_m10 | : | The data of the tens of minute is stored in this area. |
disp_m1 | : | The data of the units of minute is stored in this area. |
disp_s10 | : | The data of the tens of second is stored in this area. |
disp_s1 | : | The data of the units of second is stored in this area. |
mode | : |
The mode data of the clock is stored in this area.
Clock mode;1, Time setting mode:0. |
rb6ll | : | This area is used to detect the change of RB6(Setting in 0 seconds/Time setting cancellation switch). |
rb7ll | : | This area is used to detect the change of RB7(Time setting position alternation switch). |
rb7count | : | This area is used for the counter to detect that RB7(Time setting request switch) is ON in 2 seconds. |
digit_posi | : |
Time setting position data is stored in this area.
Tens of hour:0, Units of hour:1, Tens of minute:2, Units of minute:3. |
digit_posiw | : | This area is the workarea to calculate a time setting position. |
digit_save | : | In case of the time setting mode, a figure in the corresponding position is controlled in the blink. This area is used to store display data temporarily. |
digit_blink | : | This area is used as the counter to make blink time in the time setting mode. It counts 20 milliseconds 10 times to make the blink time of 200 milliseconds. |
blink_cont | : | The ON/OFF condition of the LED is stored in this area in case of blink control of the time setting mode. |
change_st | : | This area is the condition management area to detect up/down by the rotary encoder. |
change_wk | : | The rotary encoder input(RB4,RB5) is stored in this area temporarily. |
seg7_ha | : | A head address of the 7 segment LED control data table is stored in this area. |
seg70-7b | : | 7 segment LED control datas are stored in these areas. |

The environment designating and others
As for LIST and INCLUDE directive and Program start, refer to "Light controller".
The following specification is done as configuration word.
Oscillator | : | HS |
Watchdog Timer | : | OFF |
Power-up Timer | : | enabled |
Low Voltage ICSP | : |
OFF
(RB3 can not be used for the input/output port when not making this OFF). |
The result is 3F72h.

Initialization processing
;**************** Initial Process *********************
Port initialization
The RA port of PIC16F873 combines Analog input and Digital input/output. To use RA port as the digital input/output mode, the digital mode must be specified by the ADCON1 register. RA, RB and RC ports are set to the appropriate input/output mode.
Initialization of the LED display interval timer
Timer0 is set to 1 millisecond. At the circuit this time, 4 MHz are used as the clock of the PIC. So, the count up interval of Timer0 is 1 microsecond. Because the prescaler is 1:8, the actual count up interval is 8 microseconds. It is 1 millisecond if counting 125 times. Timer0 is a count up timer. The time-out interruption occurs when becoming 00h from FFh. So, the set value to TMR0 is the value which subtracted a necessary count value from 256. 256-125=131 is a set value.
Port initialization
The output state of the ports are initialized.
PORTC is used for the LED control. As for the circuit this time, an LED is turned off when PORTC is "1".
Workarea initialization
To control an LED display from tens of hour, the initializing value of disp_p is set to 6. Even if 0 is set, there is no problem. As for the other work aria, 0 is set.
When the mode data is 0, it is a time setting mode. So, after initialized, it becomes a time setting mode. Immediately after turning on the power, the operation mode of this clock is a time setting mode.
In the workarea initialization processing, the data for the 7 segment LED is set to the workarea too.
Initialization of the interruption condition
GIE, T0IE, INTE and RBIE are set.
Interruption wait processing
After the initialization processing ends, it waits for the interruption. The processing of the same address is repeatedly executed.

LED display processing
;********* LED disply Process (1msec interval) *********
As for the LED, 1 digit is controlled in the 1-millisecond interval. LED is turned off first to prevent an afterimage. A controlled position is decided by the contents of disp_p. The processing of tens of hour is rather complicated. The control of AM/PM and the control of tens of hour(display "1" or turning off) are done. In the time setting mode, when turning off data (0Ah) is stored in tens of hour area, a control isn't done. It is because turning off processing is done at the head of this processing.

Clock processing
;****** Clock count up Process (20msec interval) *******
This processing is started every 20 milliseconds by the outside clock. The following processing is done before processing which counts up a clock.
Time setting mode checking
In case of the time setting mode, it doesn't do the processing which counts up a clock and it jumps to the time setting processing.
Checking of setting in 0 seconds
In the clock mode, when detecting that a switch in 0 seconds is pushed, it makes a second display 00 seconds. This processing is done in the 20-millisecond interval. So, a maximum of 20-millisecond error occurs. I think that it is not in the problem substantively.
As for the setting switch in 0 seconds, last look checking is done. Setting processing in 0 seconds is done only in case of being OFF in the condition before detecting switch ON. Even if the switch is continuously pushed, the ON condition behind the first change doesn't influence setting in 0 seconds.
Time setting request checking
In the clock mode. when a time setting switch is continuously pushed for 2 seconds, it jumps to the time setting processing. The time of 2 seconds is make by the counter.
Before processing in the time setting, it makes a position for tens of hour, it makes 00 seconds and it makes the change detection interruption of the RB port possible. The change detection of the RB is to detect the change of the rotary encoder. RB7LL is made 1 to prevent setting position's changing when becoming a time setting mode.
Count check in 1 second
Because this processing is started in the 20-millisecond interval, it makes 1 second using the counter. The processing which counts up a clock is done when the counter becomes 1 second.
In case of not being a time setting mode, the processing which counts up a clock every second is done. As for each digit, a carry is done when becoming 0 from the maximum.
As for the clock this time, following display is done. AM11:59 to PM12:00 and PM11:59 to AM0:00.

Time signal control
;*** Time signal check
In the processing which counts up a clock, every units of hour is renewed, the checking of a time signal is done.
The time signal control is done at the following time.
AM7:00 |  | PM6:00 |
AM8:00 | PM7:00 |
AM9:00 | PM8:00 |
AM10:00 | PM9:00 |
RB1 becomes ON as the time signal signal when becoming this time and the time signal circuit works. OFF of RB1 is done 1 second later. OFF of the time signal signal is done every second.

Time setting processing
;****** Time adjust mode Process (20msec interval) ******
Time setting processing is started in the 20-millisecond interval by the outside clock.
Time setting cancellation
A time setting mode is canceled when pushing setting SW in 0 seconds.
In the time setting mode, the display of the setting position is controlled in the blink in the 200-millisecond interval. At the time of the time setting cancellation, the display has the possibility of the turning off condition. So, it is setting the data to the display area. Also, it makes the change interruption detection of the RB OFF.
Change position setting
A setting position is moved every time positional setting switch (RB7) is pushed. The position which can be set is hour and minute. It isn't possible to do setting in the second. In case of time setting, the second display is always 0. For the display blink processing, data in the setting position is saved. When the display of the position before movement is in the turning off condition, the re-setting of a display is done. After that, data in the change position is saved.
Display blink control
A setting position is controlled in the blink in the 200-millisecond interval. In case of turning off control, it should turn off all the segments of the LED but to light up, the original data must be saved beforehand. "digit_save" is an area for its purpose.

Time change processing
;**************** Digit change process ******************
A time data change is done by the output of the rotary encoder.
There are two pieces of output (A,B) in the rotary encoder. In case of the clockwise(CW) and in the case of the counterclockwise(CCW), the timing that each terminal touches a common terminal is different. In the processing this time, the judgement of the CW or the CCW is done using the status data.
Here ON is "1" and OFF is "0".
Status 0 | Detect "A" is ON and "B" is OFF. |
Status 1 | Detect "A" is OFF and "B" is ON. |
Status 2 | Detect "A" is ON and "B" is ON. |
The condition of "A" is OFF and "B" is OFF isn't managed. It is because it should understand the condition immediately before A and B become ON at the circuit this time.
A state transition diagram is shown below.
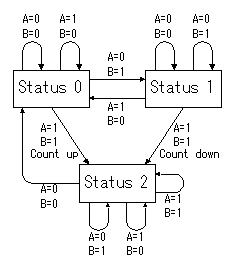
When detecting A=ON, B=ON in status 0, it increases the number of the setting position. It is CW.
When detecting A=ON, B=ON in status 1, it decreases a number. It is CCW.
A chattering is contained in the output of the rotary encoder. The chattering is the phenomenon which repeats ON/OFF when the switch becomes ON or OFF.
However, there is no problem if managing status like this time.
Relation between the signal change and the state transition when assuming a chattering is shown below.
|